React is a popular JavaScript library for building user interfaces, but managing state can become a challenge,as applications become more complex. However, Redux is a popular state management library that works well with React and provides a solution to this problem.
But,if you hire React development team then they will make the appropriate use of React state management with Redux architecture and will deliver the exceptional user experience for your business.
In this article, we’ll discuss the fundamentals of Redux and how it works with React, as well as the ideas of actions, reducers, and stores in redux.
Firstly, let’s discuss React State Management : What is it and why to use it ?
In simple words, you can say, React state management is the process of managing and updating the state of a component in React. Here the state represents the data in a component and that can change over time due to user interaction or other events.
The setState() method, a built-in method of the React component class, is used in React state management to create and update a component’s state. React automatically re-renders a component and its children when the state of a component changes.
Why do we need React state management ?
State management is important because it allows us to ensure that the data displayed in the application is consistent and up-to-date. If state is not managed properly, it can lead to bugs, inconsistencies, and incorrect data being displayed in the application. By managing state properly, we can ensure that our application remains reliable and performs well.
Now, discuss how to use React state management using redux
What is Redux?
Redux is a state-management toolkit for JavaScript programmes, inspired by Flux design. It offers a method for managing application state in a centralized and predictable manner. Redux separates the state of the application from the user interface, making testing, debugging, and maintenance simpler.
In simple words, we can say that redux was created to resolve this particular problem. It provides a central store that will hold all the state of our application. Each component can access the stored data without sending it from one component to another component.
Here is redux dataflow (how redux works).
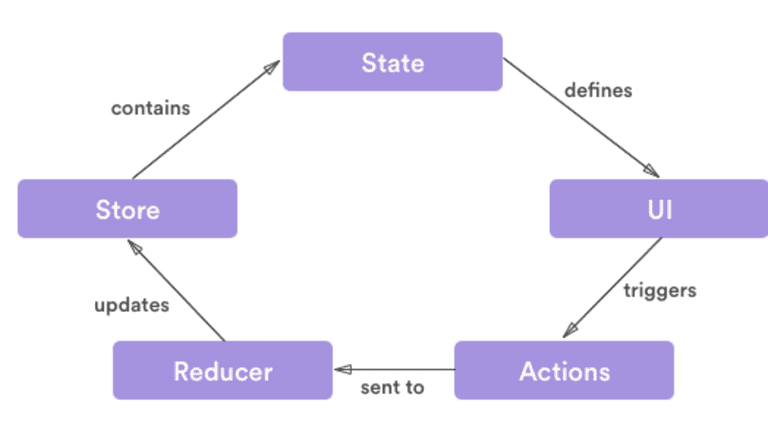
The Redux architecture consists of three main components: actions, reducers, and stores. Let’s take a look at each of them.
Actions
Actions are plain JavaScript objects that describe a change in the application state. They contain a type property that describes the type of action and any additional data that is needed to update the state. Actions are dispatched from the UI components and are handled by reducers.
Reducers
Reducers are simple functions that accept two inputs – the current state and an action – as well produce a new state. They should not modify the current state but instead create a new state object that reflects the changes requested by the action. Reducers are responsible for modifying the state of the application in response to events..
Store
The store is the central repository for the application state. It holds the current state and provides a way to dispatch actions and register listeners. The store is created by combining reducers into a single root reducer using the combineReducers function.
How Redux Works with React?
React provides a way to build reusable UI components, but managing state across these components can be difficult. Redux provides a solution to this problem by providing a centralized and predictable way to manage state.
Using the connect method offered by the react-redux package, React components can be linked to the Redux store. This function has two arguments: mapStateToProps and mapDispatchToProps.
mapStateToProps is a function that maps the current state in the Redux store to props that are passed to the connected component. This allows the component to access the application state and update its UI accordingly.
mapDispatchToProps is a function that maps action creators to props that are passed to the connected component. This allows the component to dispatch actions to the Redux store when an event occurs, such as a button click.
Let us understand using example:
In the above example,we define a Counter component that is connected to the Redux store using the connect function. The mapStateToProps function maps the count property in the store to a prop called count that is passed to the Counter component. The mapDispatchToProps function maps the increment and decrement action creators to props that are passed to the Counter component.
Conclusion
In this article, we’ve explored the basics of Redux and how it works with React to manage application state in a centralized and predictable way. We’ve looked at the concepts of actions, reducers, and stores. Actions are objects that describe a change in the application state. Reducers are pure functions that handle actions and return the new state. Stores hold the state tree of the application and provide methods to update and retrieve the state.
We also discussed how to integrate Redux with a React application by using the react-redux library. With react-redux, we can connect our components to the Redux store and dispatch actions to update the state.
Overall, Redux is a powerful and popular tool for state management in React applications, providing a predictable and scalable way to manage complex state. It requires some additional setup and configuration, but once set up, it can simplify the process of state management and improve the maintainability of our code.